Mastering JavaScript Destructuring: Simplifying Object and Array Handling
Learn JavaScript object and array destructuring. Simplify complex data handling, boost code efficiency, and master the ES6 feature
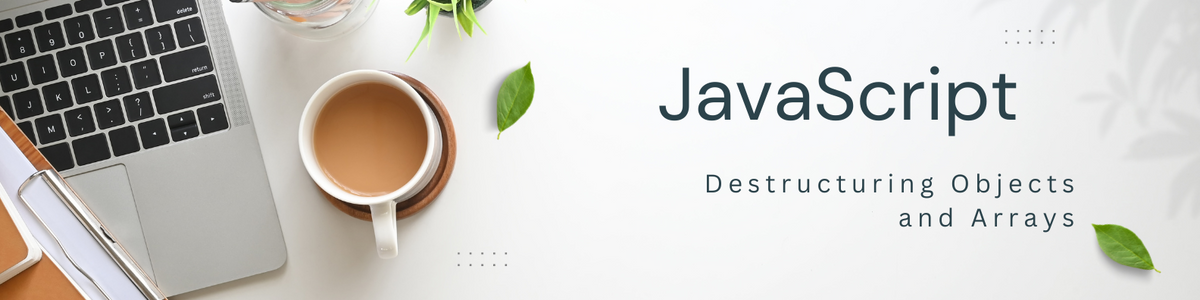
When it comes to navigating the complexities of dealing with selective properties from API responses, JavaScript offers a handy solution known as object and array destructuring. Destructuring is a streamlined and efficient technique in JavaScript for extracting values from objects and arrays. It made its debut in ECMAScript 2015 (ES6), aiming to simplify the process of breaking down intricate data structures into manageable components. This simplification greatly enhances our ability to work with object properties and array elements.
Demystifying Object Destructuring
When you destructure an object, you can effortlessly unpack its properties and assign them to variables. This proves invaluable when dealing with objects containing numerous properties.
Let's begin with a fundamental example of object destructuring:
const person = {
name: 'John',
age: 30,
country: 'Germany'
};
const { name, age } = person;
console.log(name); // John
console.log(age); // 30
In this case, the variables name
and age
are assigned the corresponding values from the person
object. It's essential to name the variables identically to the field names in the object from which you're extracting properties.
The Power of Array Destructuring
Beyond objects, destructuring is also a valuable technique for extracting elements from arrays. Array destructuring in JavaScript provides a versatile and efficient means of accessing individual elements in an array. This approach promotes code that is both concise and readable, allowing developers to manipulate array elements directly, without relying on their indices.
Here's a basic example of array destructuring:
const colors = ['red', 'green', 'blue'];
const [firstColor, secondColor] = colors;
In this scenario, firstColor
is assigned the value 'red'
, and secondColor
is assigned the value 'green'
.
Array destructuring goes beyond sequential extraction; it can utilize the rest parameter (...
) to collect remaining elements into a new array:
const numbers = [1, 2, 3, 4, 5];
const [firstNumber, ...remainingNumbers] = numbers;
Here, firstNumber
holds the value 1
, and remainingNumbers
holds [2, 3, 4, 5]
.
This syntax isn't just succinct; it's also flexible, allowing you to assign default values to variables when array elements are undefined
. For example:
const [a = 5, b = 10] = [1];
console.log(a); // 1
console.log(b); // 10
Array destructuring is an indispensable feature in JavaScript, offering developers a cleaner and more efficient approach to handling complex arrays.
Unlocking Advanced Destructuring Techniques
While we've covered the basics, there are advanced destructuring techniques that provide even more control and flexibility:
Default Values:
In JavaScript, during destructuring, you can assign default values to variables if a property doesn't exist or its value is undefined
.
const { x = 10, y = 20 } = { x: 5 };
console.log(x); // 5 - x exists in the object
console.log(y); // 20 - y is undefined, so the default value is assigned
Variable Renaming:
You can rename variables during destructuring if the property names in the object are unsuitable or conflict with existing variable names.
const person = { fName: 'John', lName: 'Doe' };
const { fName: firstName, lName: lastName } = person;
console.log(firstName); // John
console.log(lastName); // Doe
Nested Destructuring:
JavaScript allows for nested destructuring, particularly useful when dealing with complex nested objects or arrays. You can destructure properties from nested objects or arrays with ease.
const student = {
name: 'Jane',
scores: {
maths: 90,
science: 80,
english: 70
}
};
const {
name,
scores: { maths, science, english }
} = student;
console.log(name); // Jane
console.log(maths); // 90
console.log(science); // 80
console.log(english); // 70
Adding New Array Elements
In JavaScript, you can easily add new elements to an array using the spread operator (...
). This powerful technique allows you to merge arrays effortlessly. Here's how:
const oldArray = [1, 2, 3];
const newArray = [...oldArray, 4, 5];
console.log(newArray); // [1, 2, 3, 4, 5]
By spreading the oldArray
and appending 4
and 5
, we create a new array newArray
with the added elements.
Adding New Attributes to an Existing Object
You can also add new attributes to an existing object using the spread operator. This is particularly useful when you want to extend an object without mutating the original. Here's an example:
const originalObject = {
name: 'John',
age: 30,
};
const extendedObject = {
...originalObject,
country: 'Germany',
};
console.log(extendedObject);
// { name: 'John', age: 30, country: 'Germany' }
In this case, we create an extendedObject
by spreading the originalObject
and adding a new attribute, country
.
Updating Existing Attributes in an Object
The spread operator is also handy for updating existing attributes in an object. You can do this by spreading the original object and then specifying the attribute you want to change. Here's an example:
const person = {
name: 'John',
age: 30,
};
const updatedPerson = {
...person,
age: 31, // Update the age attribute
};
console.log(updatedPerson);
// { name: 'John', age: 31 }
In this example, we spread the person
object and update the age
attribute to 31
in the updatedPerson
object.
These advanced destructuring techniques provide developers with a heightened level of control, making value extraction more efficient and code more concise.
In conclusion, object and array destructuring in JavaScript offers a more concise and readable approach to handling complex data structures. By leveraging destructuring, developers can craft code that is more maintainable and scalable, ultimately boosting their productivity.
Let's continue the conversation. Have you tried object and array destructuring in your JavaScript projects? Share your experiences and insights in the comments below!
Comments ()